Non Blocking JavaScript, defer or async?
Loading scripts on HTML page is one of the biggest bottlenecks. It is normal that script blocks render until a file is fetched and executed. Browser is blocked to do anything useful like continue to build DOM. This is the reason why it’s often considered best practise to put script right before
</body>
By Default
<html>
<head>...</head>
<body>
...
<script src="script.js"></script>
</body>
</html>
Parsing the HTML stops while the script is fetched and executed. In case of slow networks or servers, displaying page will be delayed.
async Attribute
<script async src="script.js">
Script will be executed asynchronously as soon as it is available.
When a script has an async attribute, the file will be downloaded while the HTML document is still parsing.
Once it has been downloaded the parsing is paused for the script to be executed.
http://caniuse.com/#feat=script-async
defer Attribute
<script defer src="script.js">
A script that will not run until after the page is loaded.
The defer attribute tells the browser to only execute the script file once the HTML document has been fully parsed.
http://caniuse.com/#feat=script-defer
Common for both async and defer
Available only for external scripts, only if there is src attribute.
The script is fetched immediately.
Difference between async and defer
async: blocks parsing of the page
defer: does not block parsing of the page
async: scripts are executed in casual order, when they become available.
defer: scripts are executed (after parsing completes) in the order which they are defined in the markup.
Scripts marked defer are executed right after the domInteractive event, which happens after the HTML is loaded, parsed and the DOM is built.
CSS and images at this point are still to be parsed and loaded.
Scripts with the defer attribute will prevent the DOMContentLoaded event from firing until the script is loaded and finished evaluating.
What to use, what’s fastest?
TL;DR
Use script in the head with defer attribute.
<html>
<head>
<script defer src="script.js"></script>
</head>
<body>
...
</body>
</html>
How does it look?
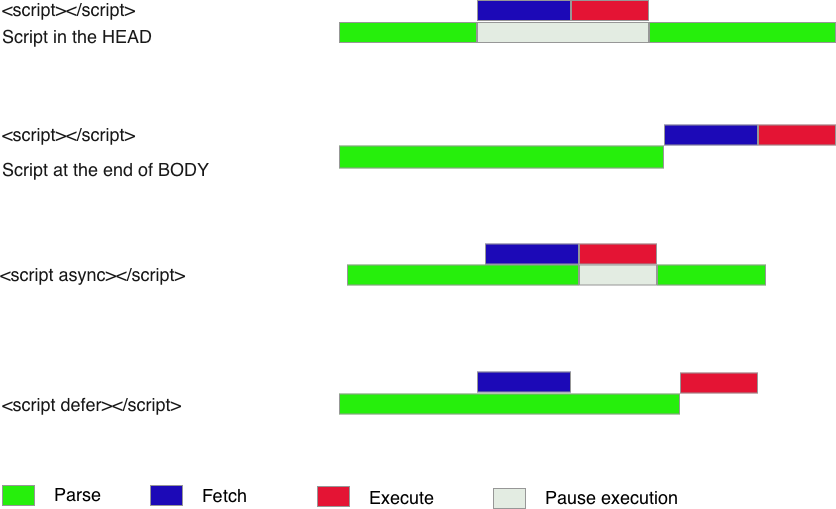
Some events
The domInteractive event
The domInteractive
event represents that the HTML has been parsed and that the DOM construction from that HTML is complete.
Browser is done processing a document, but has not yet dealt with the document sub resources like css and images.
The DOMContentLoaded event
The DOMContentLoaded
event fires when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading.
The load event
The load
event is fired when the whole page has loaded, including all dependent resources such as stylesheets and images.
Be First to Comment